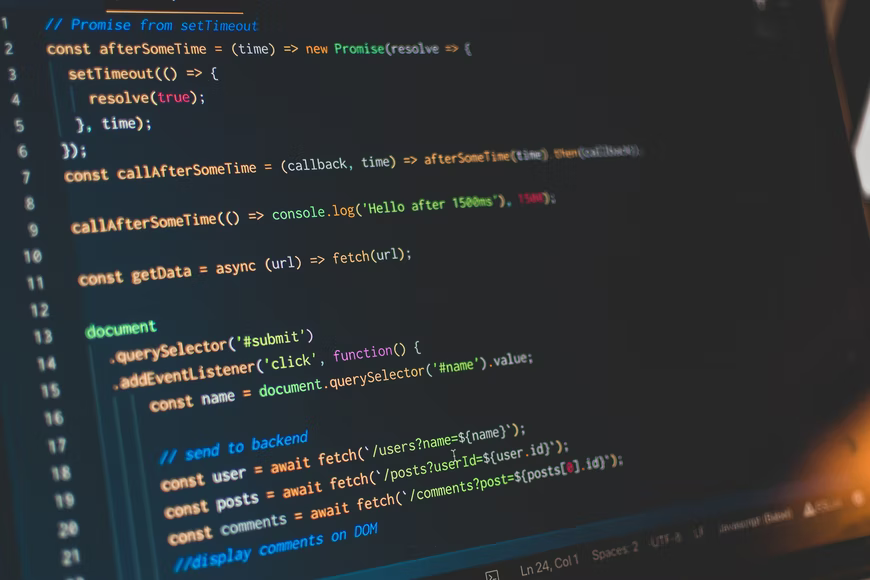
JavaScript arrays are one of the most common data structures in web development. They store a collection of values that can be accessed by index. But sometimes, you need to manipulate the array in different ways, such as filtering, mapping, sorting or splicing. That’s where JavaScript array methods come in handy.
Filter
The filter() method creates a new array with only the elements that pass a certain condition. For example, if you have an array of numbers and you want to get only the even ones, you can use filter() like this:
let numbers = [1, 2, 3, 4, 5, 6];
let evenNumbers = numbers.filter(number => number % 2 === 0);
console.log(evenNumbers); // [2, 4, 6]
The filter() method takes a callback function as an argument, which receives each element of the array and returns true or false. If the callback returns true, the element is included in the new array. If it returns false, the element is skipped.
Map
The map() method creates a new array with the results of calling a function on every element of the array. For example, if you have an array of names and you want to make them all uppercase, you can use map() like this:
let names = ["Alice", "Bob", "Charlie"];
let upperNames = names.map(name => name.toUpperCase());
console.log(upperNames); // ["ALICE", "BOB", "CHARLIE"]
The map() method also takes a callback function as an argument, which receives each element of the array and returns a new value. The new value is then added to the new array.
Sort
The sort() method sorts the elements of an array in place and returns the sorted array. By default, it sorts the elements as strings, which may not be what you want. For example, if you have an array of numbers and you want to sort them in ascending order, you can use sort() like this:
let numbers = [10, 2, 15, 4, 8];
numbers.sort();
console.log(numbers); // [10, 15, 2, 4, 8]
As you can see, this is not correct. To fix this, you need to provide a compare function as an argument to sort(), which determines the order of the elements. The compare function takes two arguments, a and b, and returns a negative number if a should come before b, a positive number if a should come after b, or zero if they are equal. For example:
let numbers = [10, 2, 15, 4, 8];
numbers.sort((a, b) => a - b);
console.log(numbers); // [2, 4, 8, 10, 15]
Remmbering whether you sort using ‘a – b’ or ‘b – a’ can be pretty hard, well it definitely was for me. So the way I remember which one to use when I’m sorting from highest to lowest or lowest to highest is that I always look at the second letter (the one after the minus sign) and I say, that letter has to be greater than the one before the minus sign. So if its ‘a – b’ ‘b’ has to be greater so we are going from the lowest ‘a’ to the highest ‘b’. Whereas if its ‘b – a’ ‘a’ has to be greater so we are going from the highest ‘b’ to the lowest ‘a’. Hopefully, I explained that well and you understand it, if not it won’t hurt to just memorize it.
Splice
The splice() method is very versatile, its incredible. It has three functions. It can changes the contents of an array by removing elements, replacing existing elements and/or adding new elements in place. For example, if you have an array of fruits and you want to remove the last two elements and replace them with “banana” and “kiwi” instead, you can use splice() like this:
let fruits = ["apple", "orange", "pear", "grape"];
let removed = fruits.splice(2, 2, "banana", "kiwi");
console.log(fruits); // ["apple", "orange", "banana", "kiwi"]
console.log(removed); // ["pear", "grape"]
The splice() method takes three or more arguments: the start index, the delete count and the optional items to add. The start index specifies where to start changing the array. The delete count specifies how many elements to remove from that index. The optional items are the elements to add at that index.
These are just some of the JavaScript array methods that can save you time and make your code more readable and concise. You can learn more about them from these sources and if you do you will realize how powerful they actually are in comparison to the code you’d have to write if those methods weren’t available.