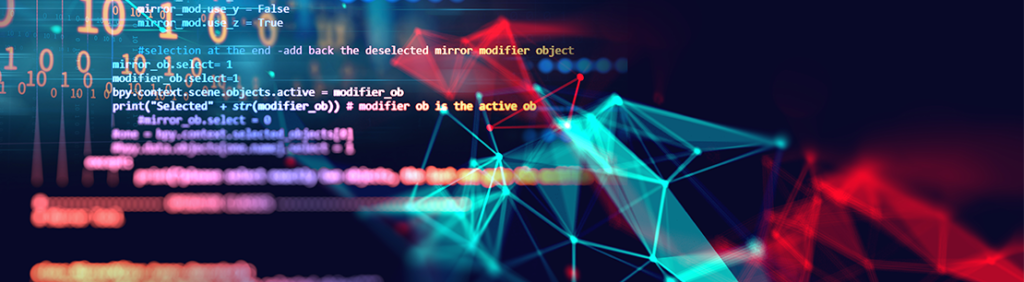
JavaScript is a great language for web development, but it can get messy as your project grows. Here are some tips to keep your code clean and organized using plain old JavaScript objects (POJOs), factory functions and ES6 modules.
Plain Old JavaScript Objects (POJOs)
A POJO is an object that has properties and methods, without any special behavior or inheritance. You can create a POJO using the object literal syntax:
// A POJO that represents a person
var person = {
name: "John",
age: 25,
greet: function() {
console.log("Hello, I'm " + this.name);
}
};
POJOs are easy to create and use, but they are not very reusable, flexible or encapsulated.
Factory Functions
A factory function is a function that returns an object. You can use a factory function to create objects with private properties and methods, using closures.
// A factory function for a person
function createPerson(name, age) {
// Private properties and methods
var greeting = "Hello, I'm ";
// Public properties and methods
return {
name: name,
age: age,
greet: function() {
console.log(greeting + this.name);
}
};
}
// Creating an instance of the person object using the factory function
var john = createPerson("John", 25);
Factory functions are more efficient and scalable than POJOs, but they can be hard to read and debug.
Factory functions are a simple and powerful way to organize your JavaScript code in a modular and reusable way. You can use them to create different types of objects with different behaviors, without relying on inheritance or complex syntax.
ES6 Modules
ES6 modules are a way to export and import values from and to different files, using the export
and import
keywords. They are useful for organizing your code into separate modules that can be reused and tested independently.
For example, you can create a file called person.js
that exports the person factory function:
// person.js
// A factory function for a person
function createPerson(name, age) {
// Private properties and methods
var greeting = "Hello, I'm ";
// Public properties and methods
return {
name: name,
age: age,
greet: function() {
console.log(greeting + this.name);
}
};
}
// Exporting the person factory function
export default createPerson;
Then, you can import the person factory function in another file called app.js
and use it to create instances of the person object:
// app.js
// Importing the person factory function from person.js
import createPerson from './person.js';
// Creating an instance of the person object using the imported factory function
var john = createPerson("John", 25);
// Accessing the properties and methods of the person object
console.log(john.name); // John
console.log(john.age); // 25
john.greet(); // Hello, I'm John
ES6 modules are a modern and elegant way to organize your JavaScript code in a modular and maintainable way. However, they are not supported by all browsers, so you may need to use a tool like Babel or Webpack to transpile your code to a compatible format. If you want to learn more about ES6 modules, you can check out this article: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Guide/Modules